The Essence of Functional Programming
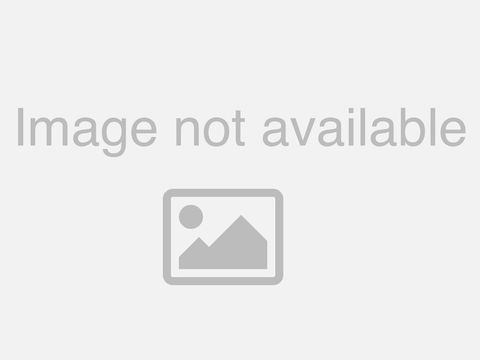
this is the essence of functional programming i'm richard feldman so we're here at functional conf 2022 this is uh from the website and as narash mentioned there are a lot of different programming languages represented here um these are some of the icons that were on the website there's even more than this but just in case you're not familiar with all the logos this is apl closure c plus plus elixir f sharp o camel haskell elm prologue scala and java and like he said there's even more at the conference now you're going to get a different experience doing functional programming in each of these languages some languages have more or different facilities for functional programming than others and of course these different experiences are going to lead to different expectations like for example if you're used to doing functional programming in haskell and you try doing functional programming in c plus plus that's just going to be a very different experience they're just very different languages likewise if you use elixir versus prolog very different experiences even programming languages that are sort of dedicated functional programming language they're not multi-paradigm at all they're just functional programming like closure and elm very different experiences and these different experiences will lead to different expectations which sometimes doesn't necessarily lead to a good experience so let me give you an example of this um so as naresh mentioned i've been working on this programming language called rock for the past couple years um it is a purely functional programming language but because performance is a big focus of the language and not just in terms of like runtime performance but also in terms of compile time performance we've been building the compiler for rock in rust and really the reason for this is that we want to go fast rust is a systems level programming language gives you a ton of control over stuff it's not really known as a functional programming language per se it's definitely like imperative at its core but there are a ton of articles about how to do functional programming and rust like tons and tons and tons of them lots of people talking about here's like a you know a blog post on how to do functional programming and rust a video on how to do functional programming rust there's even a book on how to do functional programming and rust so there's lots of people talking about how to do this and sort of uh advocating for it so um because i was writing this compiler in russ um this is actually my first time using rust for a serious project and uh i was much more familiar with functional programming i've been doing it for like 10 years and so i decided i'm going to write the parser using parser combinators so that's what i'm familiar with and since i've heard about all this like functional programming and rust stuff um i found this blog post uh from bottlesoca who is awesome she wrote a great post about how to do parser combinators and rust which i actually used to make the initial parser for this programming language and everything was going okay until i hit a problem um and this commit message uh actually this is exactly september 1st 2019 was when i ran into this problem and the commit message says attempt at making a type annotation parser so at this point i'd gotten to the form i could parse like expressions and functions and all these different things i was like okay let's get to type annotations because rock's a type check language it has type annotations and i got this weird error from the rust compiler which said recursion limit reached basically what it said is you're doing too much higher order function stuff and i wasn't built for that so i give up um just stop doing that uh i have never seen this happen in any programming language any compiler i've ever used except for this experience i had in rus and needless to say i was like well i i thought i could do all this functional programming in ross what do you mean recursion limit so kind of my my first takeaway here was this is this is just not a good experience clearly rust is not designed for heavy use of higher order functions like this i mean when you look at these like rust and functional programming and rust posts a lot of them just kind of talk about really basic examples like we're doing a map over an array or a vector or something like that um but you never really get into like what if you have a ton of higher order functions like parser combinators that's just built in this giant stack of them um russ technically didn't do that to a point but there comes a point apparently where you just reach the compiler's limit it's like no just don't do this anymore so that was my first take away my second takeaway was honestly i'm just not having a great experience trying to do what to me feels like a normal functional programming thing in rust and yet there's all these other people who have a different experience or are promoting a different idea of like yeah you should do functional programming for us that's a good idea you know rust heart lambda um all these things and so this this sort of is an example of us sort of talking past each other like i have in my head based on my experiences an idea of what functional programming is but somebody else who's maybe talking about functional programming rust they might have a totally different idea of what functional programming is that maybe doesn't involve price or combinators or anything like it so this is where these sort of different experiences that we have from doing functional programming in different languages can lead to different expectations and maybe talking past each other and miscommunications like this i kind of wish in retrospect that i didn't have this idea in my head that russ was a functional programming language or was the language where it's a good idea to do functional programming because based on my idea of functional programming i was like you know what um i kind of wish that i hadn't had that idea i'd just embrace the imperative aspect of russ because i think it's quite nice for using doing comparative programming and rust but with my elm background this this did not set me up for success so in this talk i want to explore though like what is the common ground between all these like we're at functional conf these are lots of different languages but we're all talking about similar kinds of ideas and what i want to try and do is explore this idea of like what's the essence of functional programming what's the minimal sort of essential like irremovable part that at that point we are doing functional programming no matter what language we're doing it i'm going to explore this in sort of three angles first i want to talk about what are the minimal language features required to do functional programming second i want to talk about the relationship between functional programming and math because a common thing that i hear people talk about is that functional programming is about math or has a lot to do with math so what is that relationship and third what is the functional programming style like regardless of what language you're doing it in what is the actual fp style okay so let's start about the start with the minimal language requirements in terms of features so a common answer to this question that i hear in terms of like what is the actual minimal thing that you need to do functional programming is lexical closures now this is uh an example of a first class function as a lexical closure but you might not be familiar with exactly what that term means so let me go into sort of an example of what it is so here we have an inner class in java this is an example that comes out of a blog post when they were announcing lambdas being introduced in java 8. and in this blog post this is on oracle.com it says although possible functional programming in java was by any account obtuse and awkward and what they're talking about is basically code like this where you're using this inner class in order to define something that with the lambda syntax could be defined like this much more concisely now worth noting that semantically these two actually do the same thing in java like the inner class even can do the closure thing where it captures stuff from the outer scope basically the difference here is is largely syntactic sugar like you could like like the quote says it was possible to do functional programming in java using this style of like instantiating a new runnable and making a method called run inside of it but it wasn't very pleasant um it required a lot more code than certainly the uh the lambda syntax sugar ended up um requiring so fp in java has always been possible but it hasn't always been ergonomic and now with the introduction of the lambda syntax it's more ergonomic let me give you another example of uh of where fp is possible but not necessarily ergonomic this time using a sort of different definition of a lambda a different style of closure i'm going to use sort of javascript syntax for this just for familiarity because i think most people know javascript let's say i say suffix equals exclamation point and then i write a function called exclaim which takes a string and all it does is it returns that string plus that suffix of exclamation point so in javascript this this function would work the way you expect if you call exclaim passing a string it'll return it with an exclamation point on the end but now let's say that when i was calling that this exclaim function i just happened to also put suffix like ahead of that in the same scope um let's pretend that we have like a var or a const or something like that or a let in front of both of these suffixes so this is not about global variables this is let's assume locally scoped and then i call exclaim in the same way so in a language with uh lexical scope lexical closures this will return hello world with a question mark even though in the second example we said suffix equals question marks if that had a letter or a construct bar or something like that in front of it it would be locally scoped and it would not affect this you know exclaim function but there's another style of closure where it would not do that where it would actually use the suffix that's closer to the call site rather than the one that was close to the definition site and the difference between these is that the hello world the exclamation point is lexical scoping where what matters is what was sort of lexically that is to say in the source code around it and then the other style is dynamic binding which is basically it's it's all about what was in scope around the call site so this exclaim function is actually going to evaluate suffix from the call site or what's near the call site rather than definition site like we might expect so both of these work but one of them is a lot more ergonomic than the other there's a reason that basically every language does lexical binding these days but you might be surprised to hear about a particular programming language that used to do dynamic binding so this is a talk by david turner who is the creator of the miranda programming language which is the basically the immediate presenter to haskell it's like one of the earliest languages to do lazy functional programming um and he talks all sorts of about all sorts of history it's a really nice talk i definitely recommend watching the whole thing um but one of the slides that he focuses on is he talks about some myths about lists pure lisp never existed it had assignment and go-to that is to say mutation and like literally go to like jumping uh to an arbitrary point in the source code before it had conditional expressions and recursion as far as i know lisp was actually this is from 1958 was the original language that introduced conditional expressions like if then else that actually evaluates to a value and the first language to introduce recursion um he goes on to say lisp was not based on the lambda calculus despite using the word lambda to denote functions it was instead based on first order recursion equation which is uh from the mathematician claiming so basically he's saying that you know although we talk about lambda calculus a lot we're going to talk about it some more later on the talk um lisp itself was not actually based on it and in fact the creator of lisp although he had heard of lambda calculus and knew about the term lambdas from it he actually had not really studied it and he did not base lisp on it um the m language which was part of lisp was was first order but you could pass a function as a parameter by quotation i.e is the s expression for its code basically what
he's saying right here is that list original list did not have higher order functions in the sense that we typically associate them with today what you could do if you wanted was you could basically take the source code of that function like a string and pass it in and eval that which you know if you've ever heard of eval language like javascript or something like that probably alarm bells are going off like that's not a good idea that's not a good way to do higher order functions or to simulate them but that was what you would have to do in original lisp and then he goes on to say this gives the wrong binding rules for free variables dynamic instead of lexicographic so that's where our example previously of dynamic binding comes from if you imagine if you're just basically copy pasting that code in there and then evaling it yeah it's going to run using whatever's in scope from wherever you copy pasted it because it doesn't know about what was originally in scope all it knows is the string of the source code that's where dynamic binding comes from and yeah the ergonomics are not as nice as lexical binding he then goes on to sort of give an example of this and then finally he notes not until scheme which is in 1975 uh did versions of lists with static binding aka lexical binding appear and today all versions of lists are lambda calculus based so this is really interesting because we've been talking about this sort of like you know functional programming being possible but not ergonomic i would argue that dynamic binding is another good example of this like yeah we had lambdas they were even called lambda isn't this but the ergonomics back in 1958 we're not great they're really not what we would associate with like functional programming today with higher order functions if you said here's a programming language and the way you do higher order functions is you pass a string of the source code into it and then eval that in the middle of the function i don't know how many people would say yeah that feels like functional programming to me now here's the question what's the difference between this unergonomic way of doing functions in lisp in 1958 and this other unergonomic way of doing functional programming in java in 1995. i mean both of them yeah you can write the same kind of stuff i mean arguably the java one java inner classes did actually have lexical binding so in some sense you could even argue that the java version of this was more ergonomic for functional programming despite being more verbose than the lisp version because at least it got the binding right something to think about okay this is this is kind of the strangest part to me is to realize that both java and lisp got lexical closures 15 plus years after the initial release of the language like people give java a lot of hash for you know having taken so long to finally get lambdas i mean lisp took that long together with us i mean not not exactly the same amount of time but like 15 plus years after the initial release before they got it before a scheme came out in lisbon before lambdas landed in java 8. so school closures are definitely ergonomic for functional programming but they're not required you can do functional programming without it you certainly could do functional programming in original lists i think a lot of people would agree with that some people including wikipedia as we'll see later consider lists to maybe be the first functional programming language ever but they're not essential they're not required you can do it without them it's just the ergonomics are going to be worse and honestly the same thing is true of first class functions i mean we saw from the inner class example in java that was not a function that was an inner class that had a method inside it was not a function and yet you could do functional programming in java before it got lambdas just ergonomics were not as good and in fact you can go even further with this you can do functional programming in c this is an article about and this is not just somebody who is doing like a toy project and was like i want to see if i can do functional programming and see no he was actually talking about i had a real problem and i used my experience in functional programming to solve this problem and help make my code better in c and you can go even further than that here's a stack overflow talking about functional code in assembly like pure functions in assembly language and that's even more bizarre because if you think about it assembly doesn't even have a concept of functions it's just machine instructions so like how can this possibly be well again i come back to do you actually need literal first class functions to do a functional programming style i mean if inner classes are sufficient in java what's the difference between that and a raw function pointer i mean the answer is there's no capture but again lisp didn't really have capture so do you necessarily need that i think the answer is no i think even if you don't have the most basic thing the word function is the first word in functional programming but you actually can do that style of trying to write pure quote unquote functions even if you don't have that as a primitive if all you've got is a raw function pointer which is just an address to jump to in memory it still can help you out in the same way that the functional programming style can help you out in java pre-lambdas and in original list so yeah i would say even functions are ergonomic functional programming but still not required they're not essential conceptually yeah conception you have an idea of functions but are they required to be in the language for you to do a functional programming style i think the answer is no okay so what are the minimal language features required to do fp i think it's none not even functions any programming language can do functional programming i claim and if that's true then certainly none of these other programming language features are required either to do functional programming like immutability not required pattern matching not required macros i mean all these things purity laziness these are all things that are somewhat associated with functional programming languages and you might say that these sort of feel like functional programming if that's what you're used to but if you used a programming language that didn't have any of these and not even functions i claim you could still do functional programming at least from a stylistic perspective and get the same kinds of benefits that the c programmer got when he applied functional programming techniques to his c code how granted they do certainly improve ergonomics uh when you have access to nice language features i'm making a pure functional programming language because i think that that having a language level support for uh functional programming is a great idea but i have to acknowledge that even as someone who's that committed to functional programming languages who's been using them exclusively my career for the past five plus years um they're not required for functional programming okay so having said that i would encourage you to explore the different ergonomics of different languages like if you've only ever done functional programming in one or maybe two of these languages try another one see what it feels like you might actually find that you like it more especially if you've never had the experience of using a language that was designed from the ground up to be a functional programming language um the ergonomics are pretty much across the board going to be better if you're using a language where this was the idea this was the plan rather than a language that sort of much later ended up deciding that oh we should actually add some functional programming language features even though that's not what the language was originally designed to do okay so that brings me to what is the relationship between functional programming and mathematics so this is another thing that people talk about a lot i ended up learning a lot about this uh when i was trying to answer the question what's the original definition of functional programming i wanted to just say like okay surely there was a time when no one used the term functional programming and then at some point somebody used it for the first time and then now today it's sort of part of our shared lexicon so what was that first moment like what's the earliest documented example i can find of someone using the words functional programming so i thought it might be alonzo church who created the laminate calculus back in the 1930s because a lot of times when people talk about functional programming they'll sort of hand-wave you say you know functional programming originated with the lambda calculus um and if you look on wikipedia talking about the lambda character it says it influenced the design of the list programming language yeah not really true uh it includes the design of like later lists not the original list and functional programming languages in general which i certainly think is true but did alonso church in the 1930s actually use the words functional programming i cannot find any evidence of this um i look through some of his early papers i don't see that in there i think that came later even if flame to calculus was obviously very influential in functional programming i don't think it was the where the term originated um next place i looked uh was john mccarthy creator of lisp in the 1950s so about 20 issue years later wikipedia says lisp implemented in 1958 was the first dynamically typed functional programming language i think that's a reasonable claim i'm not going to try and debate like was it or was it not the first functional programming language some people say it is some people will say it isn't um it is weird i i think if you want to be consistent though you should say that functional programming does not require electrical closures if you think that or being based on the lambda calculus if you think lisp is the first functional programming language but regardless did john mccarthy use the term functional programming again i can't find any evidence of this um i have not seen it in his early like 1950s papers about you know lisp and symbolic execution all that stuff um can't find it the first example i can find of the words functional programming being used in a paper was by peter landon who created lexical closures uh in the 1960s he wrote a paper uh that used this term um he's a british computer scientist one of the first to realize that lambda calculus can be used to model a programming language um definitely has strongly influenced by lambda calculus as we will see um as far as i can tell he was the first person to write down the the term functional programming in like an academic paper um it was this one it was called the next 700 programming languages and it's from 1966 um we can already see in this paper like a lot of things that are familiar to us today in terms of functional programming he's describing a language which we'll get to in a second talks about you know it's oriented around expressions rather than statements does that sound familiar um a non-procedural aka purely functional subsystem of this language so he's actually talking about not just functional programming but like pure functional programming with no side effects as part of this language um this is where he he talks about functional programming um he uses the phrase for the first time functional programming in a sentence in which he's actually talking about syntax which i thought was kind of funny has little to do with functional notation um he goes on to talk about uh he references another paper he talks about a correspondence between x and church's lambda notation basically talking about like the relationship between lambda calculus and mathematics and similar concepts in uh computer programming the name for his language that that he's describing here is called i swim which is short for i see what i mean which i also thought was kind of funny because here i am trying to find the original authoritative definition of functional programming and all i get is this sentence which doesn't define it it just sort of uses it and then it's in the context of a programming language called yeah let's see what i mean see what i mean yeah uh and so um unfortunately i i did not end up finding an authoritative definition here i just found this this usage of it where he just kind of casually drops it in there as if the the reader already knows what it means so my conclusion is like what's the original definition of functional programming maybe there isn't one it just kind of seems like this colloquialism that people were talking about at the time that like peter landon knew and at some point he wrote this paper and used this term that people were colloquially using and you know what i bet they all probably had different ideas about exactly what it meant so maybe it's always been this way maybe functional programming has always been this sort of vaguely shared understanding of related ideas between programmers i think that's the most plausible answer so to sum up what i ended up sort of learning in the context in the course of exploring the the origins of the term functional programming first of all it definitely referred to mathematical functions like the functional and functional programming like all these papers from around this time they're all talking about math lambda calculus mathematical properties and wanting programming to try and sort of like get some of those benefits from mathematics or steal some ideas from there so definitely that's where the function uh the word function comes from is mathematical functions um secondly um i learned you know like lisp is actually not coming from uh a lambda calculus background but it is coming from clint's ideas on functional uh recursion as in mathematical function recursion um so even more math influence on the whole like list branch of things and then i swim and which influence ml and haskell and all these other languages um definitely inspired by lambda calculus and then eventually lambda calculus ended up getting into the list family through scheme and uh and on and on it goes so definitely there is a very very strong undercurrent of mathematics sort of like that's bound up in the origin story of functional programming so what about category theory i mean quite often i've heard people say if you want to get into functional programming you're going to have to learn some category theory is that true well um so in python uh you have a concept of lists like a you know another language that's called an array but python they're known as lists um and when python programmers are talking about lists they say lists um haskell also has lists but it's quite common that you'll hear haskell programmers talk about the list monad instead it's the same idea they're both talking about lists you know a data structure called list in their respective languages um the difference is that you know colloquially haskell is referred to as the list monad now in both languages like python lists and hassellis are both monads it's like that's just a true fact about them it's really just there's a cultural difference about how they're talked about now part of this granted is that haskell has monad as part of its standard library it's really difficult to use haskell completely without learning what a monad is it's very easy to learn python without whatever that is even though both of them have monads so um is that required well no i mean an elm for example which is also a pure functional programming language like haskell um statically typed type inference a lot of similarities between element haskell but culturally and elm we just call it lists um that's totally fine uh similarly in the rock programming language that i'm working on so just called lists um you know monads don't appear in the standard library there aren't really any category theory terms it's just a cultural thing and actually like if you look at the the breadth of functional programming whether people are doing like the functional programming style or it's just a functional programming language that's not haskell or a descendant of haskell you really actually don't find that much category theory terminology um there really is just kind of like a niche of functional programming that tends to use that terminology but again like you can find different experiences no matter where you look um there's some overlap between mathematicians and programmers and some overlap between category theory terminology usage and programmers and mathematicians some people are two of these three some people are three of these three but plenty of people are just one of these three and they're interested in programming but not math and not category theory and that's fine all of those are fine so i would say based on what i've learned i don't think that category theory is required for functional programming um but it's something that you can learn if you want to and in fact it's also something you can learn if you have no interest in programming at all um they're just kind of separate and culturally you may find that it's more of a thing especially in languages where category theory terminology appears in the standard library like in haskell but there's plenty of other functional programming languages that don't have it so if it's not something you're interested in learning you don't really have to okay and finally this brings us to the functional programming style so what's the common ground stylistically between all these languages well so far from what we've explored it doesn't actually seem that they all have like some particular set of features that they all need to have not even functions that doesn't seem to be a requirement also although it seems like math is like an integral part of the origin story of functional programming i really can't find any evidence that math is sort of like part of the definition of it so it's not like you need to have any particular math background or usage of math in order to do functional programming in any of these languages so what's the common ground between them like what are you going to find is sort of the common thread i expect uh with like the talks you're going to see at this conference using more pure functions i think that's really kind of what it comes down to so pure functions if you're not familiar with the term um basically have two rules if you give them the same arguments they have to return the same return value no matter what and they can't perform any side effects along the way i really like my favorite analogy for pure functions is that pure functions are basically they're not exactly but basically lookup tables so for example let's say we have a function called string length and if i call it passing the string hi it returns two if i pass functional it returns 10 if i pass conf 2022 or returns 9. this could be implemented this function could be implemented internally as a lookup table like whatever string you give it it's going to just look up the answer in the lookup table and return it that's it no side effects and no matter what arguments you give it it's always going to return exactly the same answer so if you wanted to you could take the body of this function whatever is actually in there and theoretically you could return it replace the entire body of that function with a gigantic lookup table for like all the possible strings it could possibly accept now okay that's like assuming unlimited system resources obviously in practice you would not actually be able to implement it that way but the idea is that any pure function should in theory given unlimited resources be able to replace its entire body its entire implementation with a gigantic lookup table if you can't do that you don't have a pure function that's the rule and really if you think about it mathematical functions work the same way mathematical functions don't have side effects and and certainly if you give them the same arguments they're expected to give the same return value every single time okay so actually i mean true functions are in some sense like mathematical functions and certainly based on the history we've learned it seems like that's what people wanted them to be more like i mean that's that was sort of the goal is to get more mathematical properties out of programming um but there is one important difference between pure functions and mathematical functions which is they can crash uh system resources as it turns out are finite so you can get for example a stack overflow they can also hang like they can run indefinitely and not terminate that's also a bummer um so this is a little bit awkward um and there's this sort of natural question that arises from this which is like can computers do arbitrary math i mean can we ever get to pure functions being equal to mathematical functions unfortunately the answer is no um and to prove this to you just here's a thought experiment let's imagine that you were thinking of the biggest number you can conceive of just digits and digits and digits for ages huge huge number now raise that number to the power of itself over and over and over again as many times as you can imagine the resulting number will be so big that even if you don't do any operations on it it can be represented in the universe not just on your computer but like in the entire if you repurposed every all the matter in the entire universe and all you were trying to do with all the matter in the entire universe was represent this one number you can't do it it's too big it won't fit in the entire universe math unfortunately is infinite even just that one number is not representable let alone any functions that might involve that number is like a constant inside of it so can we ever get to a point where functions running on a computer are as powerful as mathematical functions in other words can we ever get to a point where there exists no mathematical functions that cannot be modeled by computers unfortunately not i mean computers can never have true mathematical functions in the sense that there will always exist a mathematical function that cannot be represented by a computer because math is infinite and the physical universe is finite and computers live in the physical universe unfortunately so we can sort of asymptotically approach this at best but we can never quite get there okay so one of the things that pure functions can do is they can crash this is unfortunate but there's also some other asterisks in there that we just kind of like hand wave away when we're talking about pure functions versus mathematical functions like technically they affect memory when they run like they allocate memory on the stack or on the heap um and we don't really consider that a side effect even though that is observable within the system i mean if you have another you know another function running that's like not a pure function it's going to be able to observe that memory so we kind of like to say like okay but for our purposes we're not going to treat that as a side effect and yes we know they can crash and they can you know not terminate they can run out of memory but all of those all of those aside um we're just gonna you know otherwise treat them under the assumption that those things like won't happen or that the changing of memory won't matter um also as we've seen earlier they don't really even have to be functions i mean mathematical functions yes or functions but we can get kind of the same benefits with much worse ergonomics even if we don't have access to lexical closures or something like inner classes in java or even functions themselves okay so putting all this together i'm going to claim that the functional programming style basically boils down to using more pure functions which in practice means avoiding mutation and avoiding side effects and you can do both of these even if you don't actually have access to functions this i claim is the functional programming style avoid mutation and avoid side effects and so what's the common ground between all these different things that you'll see at the conference i think it's avoiding mutation and avoiding side effects so to give an example of this um and sort of the difference in ergonomics here let's talk about like functional programming in javascript it's actually what i got into first okay it was coffeescript but i know a lot not a lot of people know about coffeescript anymore so let's just pretend it's javascript close enough basically the same language in a lot of ways um and and comparing that to functional programming in haskell let me tell you a quick story um years ago i was working at a different company and uh we were basically building a product and the team that we were on was really bought into functional programming we were using coffeescript again pretend it's javascript um but basically the rules that we tried to follow were like just pretend it's haskell like don't do any mutation don't do any side effects except at the very edges of the system um let's try to follow all the same rules that we would in haskell everybody was bought into this idea i had one co-worker who was used to haskell i was actually used to coffeescript so i had no prior experience in doing like functional programming in an actual functional language and so to me the ergonomics were like wow i noticed that it's a lot easier to do my programming um i find that this is less error-prone than what i'm used to but my co-worker really had a lot of trouble because he was used to haskell he was like this is so much harder for me like he actually ended up leaving the team because it was just so impossibly difficult for him to give up the ergonomics that he was used to from haskell and do functional programming in javascript where it was just such a much more hostile environment than he was used to for that style now in contrast today i work at a company called no red ink we make software for english teachers and we use elm on the front end and haskell not exclusively but we do use it quite a bit on the back end and basically uh now i know what my coworker was talking about in the past um the ergonomics difference is huge if you have access to all this stuff um doing the functional programming style in a language that wasn't designed for it is very very different ergonomically and in terms of like what it feels like day-to-day programming when you actually have access to a full language that was designed to be used in that way l and haskell are both pure functional programming languages but again lots of other languages at this conference um are you know like elixir and closure and stuff like that are dedicated functional programming languages that are not pure functional there's lots of different ways to go with this but the point being if you actually have a language that's built on this the ergonomics are very very different by the way if that sounds interesting to you if you'd like to use pure functional programming language to work we're hiring um okay so i claim that avoiding mutation and side effects is sort of the uh the common thread between all these this is the functional programming style but the ergonomics definitely may vary depending on the language and its level of support for that okay so summarize all the things we talked about um building this programming language called rock it's a purely functional language compiler is written in rust um i had this expectation based on all these things i'd seen about functional programming and rust that oh this is going to match with my idea of functional programming it's going to feel just like elm but really it turns out that we were to some extent talking past each other i mean all these articles that we're talking about functional programming and rust they have a different mental model of functional programming and especially the corresponding ergonomics around that that i did and i ended up kind of getting burned by that and having an unpleasant experience when i tried to apply functional programming techniques like parser combinators that i was used to in rust um so these different experiences you know can lead to different expectations and you're going to find a lot of different uh expectations and experiences at the different talks that you hear in the conference and so you should be aware of that you know what when the speaker is talking about their experience of functional programming it's probably going to be different than your experience and probably different than the other speakers experiences you should keep that in mind as you're listening to every talk we talked about so sort of these looking at this from these three different angles so what are the minimal features required to do functional programming as it turns out none not even functions it's possible to do a functional programming style even in assembly language with terrible ergonomics what's the relationship between functional programming and math definitely there is a very strong historical influence this seems to me to be where the term functional programming came from was people looking into the relationships between mathematics and programming and pure functions themselves are definitely based on mathematical functions the function in functional programming comes from mathematical functions as far as i can tell and finally what is the functional programming style it's avoiding mutation and avoiding side effects of course the ergonomics may vary depending on which language you're you're doing that styling so what is at the end of the day the essence of functional programming i think it's avoiding mutation and avoiding side effects thanks very much
2023-04-10 16:46