Python Selenium Tutorial - Automate Websites and Create Bots
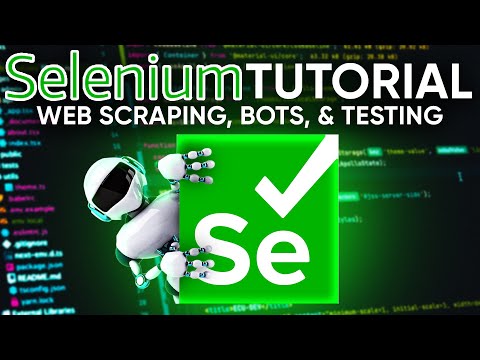
in this video I'll teach you everything you need to know about selenium to get up and running as fast as possible even if you're a beginner python programmer you can follow along what I'm going to show you is actually pretty simple yet quite powerful when it comes to creating bots or doing web automation with python I'll show you how to navigate elements click on elements send Keys hit the enter button do a bunch of stuff and I'll even run you through an entire example of automating a website from the start to the finish so you have no doubts how to do that by the time you finish this video with that said let's go ahead and get started so to begin I'm going to assume that you have python installed on your system and you have some kind of editor or IDE in my case I'll be using vs code so please open up your editor if you don't already have one you can download one for free you can simply Google VSS code and download the editor what we'll do is make a python file in my case I'll just call this main.py and now that we have this open up we're going to go ahead and install selenium what I will do is open up my terminal here for this command to work you need to have python installed and you're going to type pip install and then selenium like this if you are Mac or Linux you're going to do pip three install selenium and notice that for me I actually already have this installed so went pretty quickly again you can try pip 3 install selenium and if that doesn't work python hyphen M pip install selenium or Python 3 henm pip install selenium if none of those work I will leave two videos on the screen that show you how to fix your pip command so you can get selenium installed now that you have selenium installed we can import it and start writing a basic script so at the top of our code we're going to write from selenium import web driver don't worry if you get a yellow squiggly line that just means it's not finding the installation but it will work when you run the code we're also going to say from selenium do web driver. Chrome . service import with a capital S service and what we're going to do now is set up our Chrome driver service which I'm going to talk about more and then use that to initialize our web driver now the way that selenium works or at least the way we're going to use it is we actually create something known as a web driver which we need to download and install in just one second which will be an automation tool that controls Google Chrome now you can do this with Firefox and a bunch of other browsers but Chrome is definitely the most popular so what we're going to do is create something known as a service and we're going to say service is equal to service and inside of here we're going to pass our executable uncore path and that's going to be equal to a string we're then going to say our driver is equal to web driver. Chrome and we're simply going to pass inside of here service is equal to service now at this point in time nothing is going to work because we don't yet have the web driver that we need again this is a piece of software which will be able to control Chrome for us and kind of act as a real user as we integrate or as we kind of automate the browser so what we'll need to do now is go to a link let me pull up that link and I will leave it in the description so we can download our Chrome web driver all right so I've copied the link here as a comment and I've also put it right here inside my browser now I'll put this as a link in the description so you can just click on it and this will bring you to the page where we'll be able to actually download our Chrome web driver now we need to make sure that the web driver we download is the same version of the Google Chrome instance that we have so what I'd recommend you do is just update your Google Chrome so it's the most recent version then what you can do is go to this little link here it says at the Chrome for testing availability dashboard so it says latest Chrome driver binaries we're going to click on this and it's going to bring us and show us all of the different versions of the web driver we can download now what we're going to do is click on the stable versions for whatever the most recent version of Chrome is in this case it's 119 when you're you're watching this video it'll probably be higher than that and when I click on that it's going to bring me to the stable links what we'll need to do is look for the Chrome driver installation not Chrome and we want to install the win64 version if we're on Windows if you're on Linux or Mac obviously you're going to install the appropriate version if you are on the um what do you call it M1 M2 M3 chips I believe you need armm and if you're on Intel you do the x64 installation so I'm going to copy this link here for Chrome driver for win64 and I'm going to paste this in my browser when I do that it will just pop up a download window for me what I'm going to do is find the location where I'd like to save this so it's going to be in the selenium folder and it's going to download a zip folder for me so now that I have that downloaded you can see it actually appears here in my vs code I'm just going to open this in my file explorer and I'm going to extract this zip folder on Windows you can do that by right clicking and click on extract all and just extract the files to this location on Mac you should just be able to double click it to open or you might need to use a kind of decompression tool but I'm sure on Mac you're familiar with using a zip file if not you can always ask chat gbt or look up how to uncompress it anyways what we're going to do here is grab this chromedriver.exe file I'm just going to copy this file so contrl C or in my case contrl X cuz I cut it and I'm going to paste it so it's directly in the same directory as my python file so notice I made my python file main.py here and chrome driver is right beside it then I can go ahead and delete these zip folders okay very important that Chrome driers is in the same folder as your python file once you have that exe file uh or whatever the installation file is depending Mac Linux Etc what you're going to do is put the name of it here so we're going to say chromedriver.exe
okay so I've placed that here and now I'm specifying the path to this file is well this right which is right beside my python file again if you're on Mac or Linux you're going to have to adjust this so if it says like DMG or some other um kind of extension here you're going to replace exe with whatever the file name is that you have beside your python file I think that is fairly clear okay now that we have that we have the ability to actually grab a website so let's do that we're going to say driver. getet and then inside of here we can just put a URL that we want to go to so I'm going to say google.com just for testing purposes right now I'm going to import the time module okay and I'm just going to say time. sleep and I'm going to sleep for 10 seconds and then I'm going to say driver. quit now the reason I'm doing this is I just want to have a little bit of a delay so you guys can see what's going on you don't need to do this on your computer anyways this is all we need so once we have the Chrome driver we can do driver doget then we can go down here and say Python and then the name of our file which is main.py notice this only works when I am in the same directory where my python file is and if you know how to run your python code you can run it anyway you want this is just how I run it if you're on Mac or Linux you can do Python 3 main.py
anyways I'm going to go ahead and hit enter notice it's going to go to google.com it's going to wait about 10 seconds we'll get some logs down here and then it should actually quit the window for us let's give this a few seconds 5 4 okay and it quit and there you go that is the first instance of running selenium so we got Chrome driver set up we're able to navigate to any website we would like we're going to sleep for a few seconds and then we can quit the driver at any point we want by running driver. quit if there is no activity in the driver then it will automatically quit so I like to leave this sleep here just so we can see what's going on and actually visualize the whole process all right so now that we've done that I will quickly show you an example where we can actually search for something on Google and then we'll get into a bunch more interesting things we can do with selenium now that the setup is done so what I'm going to do is go to the top of my program and I'm going to say from selenium do web driver.
common. bu import buy with a capital B now what I'm going to do right now is show you how we search for elements in the HTML and then once we have access to an element we're able to interact with it click it change it do whatever we need to do so what I want to do now is I want to actually find the Google search field and then I want to click into it and I want to type something in it and then hit enter so we actually perform a Google search Okay how are we going to do that well to do that we will say the input uncore element is equal to our web driver dot getor element and then we're going to say bu so by which we just imported up here Dot and then we have a bunch of different options here now in my case I think I'm going to go with class uncore name we'll talk about this in one second and then we're going to specify the class name of the input field that we want to access so let me explain this a bit more in depth and how this works let me just bring open google.com here okay so anytime we are scraping a website or interacting with it with a bot what we want to do is access the HTML elements that are on the page so that we can interact with them so the way that we can figure out which elements interact with is we can rightclick if we're in Google Chrome and hit inspect when we do that it will bring up the kind of console or terminal window here whatever you want to call it if I right click on this again it kind of shows me the element in the code now what I'm looking for here is some kind of identifier that I can use to access the element now we can use the ID we can use the class name we can use the tag name there's a bunch of different ways that we can access this but in our case right now we'll go with the class so notice the class here is GF YF has no meaning to me but I'm just going to copy that class and I'm going to paste that here now since I specified that we're searching by class name what we're going to do is look for the first element on the page that has this class and then we'll access it and be able to interact with it now if we wanted to we could search by the ID as well and we can search by a few other things that I'll show you later but for now we're going to do class name now once we have the input element what we can do is actually send keys to it and we can click on it so I'm going to say the input uncore element. send sendor keys and this allows me to just type something into the element so in this case I could type something like Tech with Tim and then you'll see if we go ahead and run our code that what should happen is we actually open up Google Chrome and we type Tech with Tim however it gave me an issue here it says web driver object has no attribute get element uh okay that's weird I believe it's because it is find element not get element so let's fix that up quickly and now you should see that it types Tech with Tim here in the Google search bar okay so there you go we access the element we're able to type in now what we probably want to do here is actually hit enter so we can search so to be able to send a specific key like an up Arrow key down arrow key something that's not something we can actually type what we need to do is another import we're going to say from selenium do web driver. common Dot and I think actually
this might not be common it is common Keys import and we're going to import keys and then what I can do is simply put a plus sign here and say keys Dot and with all capitals enter there's a bunch of other Keys you can access as well like shift key Capital Etc in this case we just want enter so now what will happen if I run my code is we will open up the web browser type Tech with him and hit enter and it will bring us over here to this page sweet so that is the first example of using the web browser if you want to find an element again you can do that by the class name the ID a bunch of other things which we'll look at in a second all right so all of this is great so far however I do want to mention that it is quite common that when you're doing web scraping especially with this technique you're going to run into a lot of issues now that's because you're using a bot and a lot of companies today are pretty smart they can automatically detect Bots and they'll either just straight up ban your IP address boot you off the website redirect you or they'll give you things like captas that you need to solve that you won't be able to solve because well you are indeed a bot when you're interfering or interacting with that website now at the same time if you want to do a task say hundreds of thousands of times it's not going to be feasible to do that on your local computer you're going to want to push that out to the cloud and be able to run multiple instances of your browser all at once now fortunately the sponsor of this video bright data actually has a solution for that and 360° solutions for any type of kind of automation web scraping task Etc now I've been working with bright data for over 2 years and they have something called the scraping browser which which is relatively new now what this is is a headful browser that you can connect to from your local computer that runs in the cloud and has automatic unlocking tools that means that you can browse any website you want it will automatically solve captures for you it'll rotate the IP address so the IP will never get banned and you can browse from any location you want meaning even if you want to access something like a geolock website you're actually able to do that with bright data the nice thing is it's literally a few clicks to get it set up super easy I'm going to show you right now and if you want to start with it or try it out you can actually just get a free trial with bright data and I'll leave a link to that in the description anyways give me a second here to go to my dashboard I'll show you how we spin up the scraping browser and how we can just plug and play it right into our code so we can automatically unlock any websites or solve any problems we might run into all right so I just went to my dashboard here now as I mentioned bright data has a ton of tools and not just the scraping browser if you're trying to do anything at scale when it comes to scraping the web interacting with websites proxies Etc definitely check them out regardless I'm going to go to the view proxy products here you can see I already have a bunch of stuff that I was using previously and I'm going to go to add and I'm going to click on scraping browser which was what we're going to use now this is a headful browser and what that means it will actually work with Puppeteer playwright selenium and it has a few other features that headless browsers don't have so just something to note in this case we can just call this tutorial selenium like that go ahead and spin this up we'll give that one second to run okay and what we can do now is go to configuration and sorry not configuration we can go over here to check out the code integration and examples and we can just click on the language that we're going to use as well as the library and it will tell us exactly the code we need to get this running so I'm going to go here to Python and I'm going to click on selenium and I'm just going to copy all of the uh code that it has here because it already has all my connection parameters and everything and if I wanted to I could even go over here and I can kind of choose some things like taking a screenshot finding elements I can pick the site Etc okay so I've selected that now and I'm going to go over here I'm just going to make a new file Main to.py and paste all of this in now let's just make this full screen what this is going to do is go to let's go google.com Okay so let just change this to google.com and if I go ahead and run this code what it will do is actually spin up a browser for me go to google.com and perform any of the task that I want it'll just work out of the box with selenium so if I wanted to search for Tech with Tim if I wanted to scrape the website doesn't matter it's just going to work and you can see there's some code here that we could uncomment that actually will tell us the status of this automatically solving captas or unlocking the website for us now in this case we don't need it because there's not going to be any captas on the website we're going to but if there were it would just automatically solve them for us and allow us to move forward um kind of with the scraping so what I'll do is go to python demo to.py but before I run this I quickly just want to spin up uh something here so if I go over and back to the next page if you want to see the progress of what's Happening here since this is running in the cloud and not on your local machine you can click on this button which is the Chrome Dev tools debugger and it will actually show you in live time what's happening um kind of with the browser so you'll see if I run the code here so python oops uh what do we call this main to. p. demo to.py and
then we click on the Chrome devb tools debugger it will actually show the session and we should be able to see that it's gone to Google uh let's give this a second here yes you can see it went to Google and then obviously if something was happening here it would show us that and then every time we run this we can kind of reconnect to that session hopefully that makes sense but I'm just trying to illustrate that you're not going to get like a big Chrome tab popping up here which you actually don't want a lot of times when you're doing this at scale instead you got to go over to Bright data directly to do this anyways I wanted to show that to you because I know a lot of you will be doing this for kind of more advanced tasks so having a solution that's able to unlock the websites is super super useful now for the rest of the tutorial we don't actually need this because there won't be any captures or anything that we need to solve but if you do any web scraping at scale definitely check out bright data very costeffective solution anyways you guys can see them from the link in the description thanks to them for sponsoring this video okay so now that we know how to get by captas unlocking and all the other issues that could occur what I want to show you is a few other things we can do with selenium and I want to actually automate a website for you and show you an example of how we would start this process from the beginning to the end all right so just to continue this example a little bit before we get into the larger one I want to show you a few things that might be useful so first of all if you have an input element like a text field or a text area you can actually use this do clear method that's useful because sometimes it could have content inside there so you might want to clear it out first and then actually send some keys to it because this will not override what's in there it will just append to the field so you want to clear first and then send the keys other than that a few things we can do are actually wait for the presence of elements so sometimes what's going to happen is it's going to take a few seconds to actually load the website and that means that we'll try to maybe access an element that doesn't yet exist so if we want to wait for an element to exist what we can do is the following syntax and to do that I just need to import something going to say from selenium do web driver do what is this support. UI import web driver weight and then we're going to say the pretty much the exact same thing so copy this from selenium web driver. support we're going to import the expected uncore conditions as EC okay okay now this is going to seem a little bit complex but this will allow us again to wait for the presence of an element before we go forward so we don't run into an issue if what we're looking for doesn't yet exist so what we can do is say web driver weight and then we can pass in our web driver and we can pass in the number of seconds that we want to have as a timeout in this case I could put something like 5 seconds and this means if after 5 Seconds the element doesn't exist go ahead and just crash the program we're not going to wait for it so however long you want to wait you put here in seconds we're going to say dot and this is going to be in and then inside of here we're going to say EC Dot and this is going to be prescore of element okay and this is going to be located and then inside of here we're going to put a tupple and we're going to say by Dot and in this case we could do something like class unor name and then we can put the class that we want to wait for so what this is going to do again is it's going to use our web driver it's going to wait for up to 5 Seconds until we locate an element by its class name that has this ID now notice I do have an extra set of parentheses in here this is needed so what I could do is I could put this right before my input element and now if it was taking a second to load google.com maybe you have a slow
internet connection or something you will wait until this element exists once it exists you will then find it access it and you won't get errors saying hey we can't find this element on the page okay so that's great if we run our code that will work but another thing I want to show you is how we navigate based on some text so if we pop open our code here and sorry that's not the one that I wanted to run I want to run main.py you will see that when we search Tech with Tim we have some link text here now I don't necessarily know what the ID of this will be but what I do know is that I want to click on something that says Tech with Tim so what I can do is actually find an element based on its text especially if it's a link so if you want to click on some link that's going to redirect you to another page page you can do what I'm about to show you here so I'm going to say link is equal to driver. findor element and this is going to be buy do partial uncore linkcore text and then for this text I'm going to say Tech with Tim now what this means is that if tech with Tim in this capitalization exists inside of some kind of anchor tag or link tag we're going to go ahead and access it so this is what I'm looking for this link and then I can simply say link. click and what this will do is actually redirect me to whatever the link goes to okay so this allows us to click on a link now if you wanted to find the exact text so maybe you don't want to find something that just has Tech with Tim you want it to only say Tech with Tim you can use Link text like this this finds the exact text this will see if text is contained inside of an anchor Tech now the way that all of these find elements work is will'll find the first thing on the page that adheres to whatever you put here now for some reason you wanted to find all of the links that had Tech with Tim you could actually do find elements what that would then do is return you an array of all of the different elements that you could iterate over so for example you'd have like element one element two Etc then you can access those elements and you can do whatever you want with them so if you're trying to find multiple you can use find elements if you just want one which is most of the time what you want then it's just going to be find element okay so if I do this now it should work however we might again run into that problem where it takes a second to load the element and we get an issue here so what I'm going to do is just copy this I'm going to go here I'm going to take my buy I'm just going to paste that inside of here so now I'm going to wait for this to exist as soon as it exists I'm going to click on it and I'll wait up to 5 seconds so I'm going to run my code give this a second Tech with s and then it should actually click on this link here and notice it does it clicks there and goes to my YouTube channel okay there you are uh that is kind of the first example now that we have this what I actually want to show you how to do is automate the Cookie Clickers website let me set up that demo and we'll be right back all right so I've gone to the famous website of Cookie Clickers which was one of my favorite games when I was younger and in school anyways the point of this game is you click on this cookie it gives you a bunch of cookies here and then you can buy some upgrades which will automate the cookie collection process so you can buy this cursor upgrade for example and it should just automatically click the cookies for you you can buy all these other kind of upgrades that appear very simple though you just collect as many cookies as possible and what I want to show you how to do here is automate this site now to automate the site what we would need to do is navigate to the site we're going to have to click on this cookie infinitely so as many times as we can now once we have enough cookies to buy an upgrade we're going to actually go over and click on that upgrade to purchase it and this way I'll be able to show you how we access the value of different elements and do things dynamically on the website so this will give you a bit of a better idea of how to do a more in-depth automation obviously still pretty simple but I think this will be a good thing to look through so the URL is right here but I will leave this in the description you also can just Google Cookie Clickers and it'll pop up really easily and I've just set up the same kind of boiler plate that we had before so I brought in all my imports I brought in my service and my driver and I'm now going to this website so now what we can do is actually pop up this website so I should have left this open and kind of the first thing we want to do here is we want to start locating the classes that we're going to want access to when we're automating this site so I'm going to right click on this and click on inspect and you'll see here that we have this button and it's called big cookie and this is the ID so what I'm going to do is just copy in this ID and the first thing we'll do is we'll just look for an element that that has this big cookie ID and then we'll start clicking on it okay so we can do that as step one we can say the cookie uncore ID is equal to big cookie and then we can find this so we can say the cookie is equal to driver.
findor element this is going to be by the ID and then we're going to say comma cookie ID and for now we can simply say cookie. click now there is going to be an issue here but I want to show this because it's somewhat educational what I'm going to do is run my Cookie Clickers file I created a new python file here so I'm going to go ahead and run that and notice actually that what happens right away is we get an error now the reason we get an error is it says that the element we're trying to click is not clickable at this point and the reason we got that is because you might have noticed that when this popped up we'll see if we can look at it quickly again it actually asked us to pick a language now this is something again that could happen that might not happen in your instance because you might have already selected a language like I did so what we need to do in this instance is actually select a language once we select the language it's then going to load again and then we can start pressing on the cookie so to select the language that's kind of the first thing we'll need to do here so what I'm going to do here is just copy in this line right here because we're going to use this to wait for the presence of an element and what I'm going to show you how to use here is something known as the X path now X path is kind of this fancy syntax that you can use that allows you to search for specific text specific attributes all kinds of stuff I'm just going to copy in the exact line that we need I'll kind of explain it a bit but if you want an X paath piece of code and you don't know how to write it you can just go to chat GPT and say hey here's the HTML element I'm trying to Target or I you know you can kind of describe what it is that you need and it will give you actually the F XPath syntax sorry so again I know it seems a little bit weird we have two slashes and asteris set of square brackets and then we're using a function called contains and what we're looking for is some text so the text inside of an HTML element that contains English because this is the language we're going to select I know that's not super clear but this is X path you can look it up if you want more information kind of a cool way to select HTML elements I'm not an expert so that's going to be the extent of my explanation so we're going to wait for that language to pop up then we're going to say language is equal to driver. find okay and we're going to find by X path this language and we're simply going to click on it now to click on it we can just say language. click okay so we're
just going to click on that element and now we're going to wait for the cookie element to appear so we're going to copy this okay and we're going to go by ID cookie ID we're going to paste that inside of here and then we're going to say time. sleep 10 seconds down here so now what should happen is we will wait for the language to appear click on the language wait for the cookie to appear and then click on the cookie okay so we're going to go ahead and run this code give this a second here to load up and we got an issue here it says web driver has no attribute find okay this because needs to be find element sorry about that guys let's fix that up okay give this a second and there you go it went ahead and clicked on the language and then it clicked the cookie one time sweet now notice we have our upgrades down here so what we'll have to do is actually find um what do you call it here the classes of those upgrades so we can look at them we also need to know how many cookies we have so if we open up Cookie Clickers here you can see that first of all if we want to see the number of cookies that we have it is inside of this div so if we open this up we can go inside of here and that's not right inspect um okay for some reason reason it's having trouble telling me where the cookies are I think it might be here ah there you go so we have div ID cookies okay so that's what we want we're going to access the ID cookies to see how many cookies that we have so let's start with that we're going to say our cookies ID is equal to cookies let me just minimize this for now and what we'll do is we will set up a while loop here and we'll say while true and while this is true we're going to click the cookie and we're simply going to print how many cookies we have so that we can see what that number is so we're going to say our cookies count this is going to be equal to driver. findor element we're going to say buy doid again and then this is going to be the cookies ID okay now what we want to do is access the text like that so now what we can do is we can print out the cookies count which is is going to give us the text inside of that element and before we do that we can say cookie doclick so now we should just infinitely click the cookie and be printing out how many times or how many cookies we have so let's run this code and give this a look okay give this a second to go through here and we got an issue and it says what is the problem okay I got a weird issue there but I'm just going to remove this cookie click online 30 which I think will actually be the issue I'm going to go here and run this again and let's hope that it works this time okay give this a second and there you go now it's working and you can see it's printing out how many cookies we actually have now I'm going to stop this and notice it says 123 cookies per second zero okay don't worry about that what we want to do now is just get the number because we need to have just the number not this whole string part so to do that I'm just going to go to here and I'm going to say do split at a space and I'm going to grab the first value here then I am going to say the cookies count is equal to the cookies count do replace and I'm going to replace all the commas with an empty string and convert this into an integer the reason for that is that we might have commas in this number if you have a comma it's going to cause an issue in Python so we're going to remove all of those and convert this into an integer and now if we print out the cookies count will just give us that numeric value now that's fine I think that we understand that's going to work now we want to look at the upgrades so if I click on the upgrade here you can see that the upgrades are within this product zero product one product two and product 3 ID field and what we need to know is actually the price of the upgrade so if we go into content you can see that we have these IDs here and they say product price zero and at the same time they'll say product price one product price two product price three so what we want to do is essentially iterate or look through all of these different IDs see what the price of the upgrades are and if we have enough cookies we want to click on them so I'm going to show you the code to do that what we're going to do here is we're going to write the product price prefix is equal to product price and we're going to say the productor prefix is equal to the product like that okay now what we will do is we're going to say four I in range four because we have four products that we want to iterate through we're going to say the product uncore price is equal to the driver. find element and this is going to be the product price prefix plus the string of I and we're going to do this by do ID because they're all ided okay now what I'm saying is okay I I want to look for product price first zero then product price one then product price two then product price three now just like before I need to do this conversion so I'm going to say product price is equal to product price dot replace and I'm going to replace the commas with an empty string to remove them and I'm going to convert this to an integer and I'm going to say if my cookies count is greater than or equal to the product price then what I'm going to do is actually locate the product now the way I'll do that is the following I'm going to say product is equal to driver. findor element and I'm going to find Again by ID this is going to be the product prefix plus the string of I and I'm simply going to click this now there's a few other things that we need to do but this is kind of the basic idea we're going to iterate through all of the different products if we can afford any of those products we're going to go ahead and click on them once we click on them we'll simply break the for Loop and then we'll continue this process that means anytime we have enough cookies to be able to upgrade we'll automatically do that upgrade and that's all we need to do now a few things to note here first of all I found the price okay so once I find the price if I have enough cookies based on the price what I'm going to do is find the product the reason I need to find the product is because that's the button whereas the price is that little text tag if I try to click on the text tag it's going to give me an error I need to click on that button as a whole so that's why I have two separate finds here um that I'm looking at hopefully that makes a bit of sense now one thing that we need to do is we just need to make sure that this product price is actually a string because it's possible that it won't be so what I can do is simply say this if product price dot is digit so I'll say if not product price. is digigit then we are
simply going to continue which just means we'll go to the next product this way we don't get an issue when we try to convert it into a uh integer now I can also actually just throw the do replace up here and this reminds me I also need the text so let's say text. replace okay so I'm going to grab this element I'm going to grab the text inside of it I'm then going to replace the comma with the space so that we get nothing there I'm just going to remove that here cuz we no longer need that okay I'm hoping that's clear but this actually would be the finished code so if we go ahead and run this now we might get an error we might not let's see and figure out if this is working okay brings us over here to Cookie Clicker and we do get an error it says stale element reference okay I'm not sure what that is sometimes I think you just get a weird bug with a caching issue so if I rerun this maybe it will fix itself and there you go it did fix itself and notice it actually will automatically go over here and click on the cursor uh every time we have an upgrade so it'll just keep doing this and eventually once we get to the point that the cursor is more expensive than the grandma upgrade then we would go to the grandma upgrade and vice versa okay now you can see why I needed to remove the commas because we have like one comma 1,000 that's obviously going to be an issue and python is not going to like that so I needed to remove it so we can let this run as long as we want to but that is automating Cookie Clickers and that's pretty much all I had to show you in this video so with that said I think I will wrap it up here this code will be available from the link in the description I hope you enjoyed I look forward to seeing you in another YouTube [Music] video
2023-12-05 18:28